A modular Edge Side Includes component for JavaScript Compute
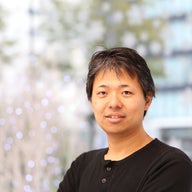
Senior Software Engineer, Developer Experience Engineering, Fastly
In the summer of 2022, my teammate Kailan worked on a Rust crate for Fastly Compute, implementing a subset of the Edge Side Includes (ESI) templating language, and published a blog post about it. This article was significant not just because we released a useful library, but because it was a brilliant illustration of what Compute can bring us: a programmable edge with modular functionality. And now that JavaScript has been generally available on Compute for more than a year, it was time we had a similar solution for our JavaScript users. Fastly’s ESI library for JavaScript, now available on npm, allows you to add powerful ESI processing to your application.
Programmability and Modularity
For almost a decade, Fastly’s CDN has had support for Edge Side Includes (ESI), a templating language that works by interpreting special tags in your HTML source. It revolves around the tag <esi:include>
, which instructs the edge server to fetch another document and inline it into the stream.
index.html |
---|
|
header.html |
---|
|
output |
---|
|
When Compute entered the scene, the edge landscape changed in two main ways: programmability and modularity.
Soon after our platform support for Rust had stabilized, we published a crate for Rust that implemented ESI, and added programmability. You could now configure, using code, how to build additional backend requests, and how to handle response fragments. You could even perform ESI processing on documents that don’t originate from the backend server. This programmability differentiated it from the ESI support we have in VCL, which was limited to the fixed set of features we offered.
At the same time, this approach was highly modular, giving the programmer a choice to perform this ESI processing on a per-request basis, and the option to combine the processing with other modules that work with compatible data types, and apply them in any order and/or logical condition specified.
Next target: JavaScript
Similar to our Rust release, we wanted this JavaScript library to be programmable. Fastly’s JavaScript support has always embraced the Fetch API and its companion Streams API. One useful feature of the Streams API is the TransformStream interface, allowing for data to be “piped” through an object in order to apply a transformation–perfect for ESI. By implementing the ESI processor as an implementation of TransformStream, we were able to fit it right into a Fastly Compute application written in JavaScript.
Here’s how you can stream through it:
const transformedBody = resp.body.pipeThrough(esiTransformStream);
return new Response(
transformedBody,
{
status: resp.status,
headers: resp.headers,
},
);
The transformation, which we call the EsiTransformStream, is applied to the stream directly, alleviating memory and performance concerns. This means:
There is no need to buffer the entire upstream response before applying the transformation.
The transformer consumes the upstream response as quickly as it can, and processes ESI tags as they show up in the stream. As the transformer finishes processing each ESI tag, the results are released immediately downstream, so we are able to hold the minimal possible buffer. This allows the client to receive the first byte of the streamed result as it becomes ready, without having to wait for it to be processed in its entirety.
In addition, this design is modular, making the EsiTransformStream just another tool you can use alongside other things. For example, you might have other transformations you want to apply to responses, like compression, and a response can be piped through any number of these transform streams, since it's a completely standard interface. If you wanted to, you could even conditionally enable ESI for just certain requests or responses, such as by request headers, paths, or response content type.
Here’s how you instantiate EsiTransformStream:
const esiTransformStream = new EsiTransformStream(req.url, req.headers, {
fetch(input, init) {
return fetch(input, { ...init, backend: 'origin_0' });
}
});
The constructor takes a URL and a Headers object, and optionally takes some options as a third parameter. As described earlier, the main functionality of ESI is to download additional templates, for inclusion into the resulting stream. Encountering an <esi:include>
tag uses fetch as the underlying mechanism to retrieve a template, and the main purpose of these parameters is to configure those fetch calls:
The URL is used to resolve relative paths in the src of
<esi:include>
tags.The Headers are used when making the additional requests to fetch the templates.
The optional configuration object can be used to override the behavior of the fetch that is made, and to apply other custom behavior, such as how to process the fetched template, and custom error handling.
In the simplest case, you’ll use just the fetch value of the configuration object. If you don’t provide it, then it uses the global fetch function instead, but in Compute you’ll need it to specify a backend for the fetch to use when including a template (unless you’re using the dynamic backends feature). The example snippet above assigns the backend named origin_0
before calling the global fetch.
That’s it! With this simple setup, you can have a backend serving ESI tags and a Compute application processing them. Here’s a full example that you can run:
Support for ESI features
This implementation offers more ESI features than others we have made available in the past.
Error handling
Sometimes, a file referenced by an <esi:include>
tag may fail to fetch due to it not existing or causing a server error. It’s possible to ignore the error in these cases by adding the attribute onerror="continue"
.
<esi:include src="/templates/header.html" onerror="continue" />
If /templates/header.html
causes an error, the ESI processor silently ignores the error and replaces the entire <esi:include>
tag with an empty string.
It’s also possible to use more structured error handling by employing an <esi:try>
block, which looks like this:
<esi:try>
<esi:attempt>
Main header
<esi:include src="/templates/header.html" />
</esi:attempt>
<esi:except>
Alternative header
</esi:except>
</esi:try>
The ESI processor first executes the contents of <esi:attempt>
. If an <esi:include>
tag causes an error, then the ESI processor executes the contents of <esi:except>
.
It’s important to note that the entire <esi:try>
block is replaced by the entirety of the <esi:attempt>
block if it succeeds or the <esi:except>
if there is an error. In the above example, if /templates/header.html
causes an error, then this also causes the text “Main header” not to appear in the output; only the text “Alternative header” is included. See the ESI language specification for more details.
Conditionals
ESI also allows conditional execution, by performing runtime checks on variables. The following is an example of such a check:
<esi:choose>
<esi:when test="$(HTTP_COOKIE{group})=='admin'">
<esi:include src="/header/admin.html" />
</esi:when>
<esi:when test="$(HTTP_COOKIE{group})=='basic'">
<esi:include src="/header/basic.html" />
</esi:when>
<esi:otherwise>
<esi:include src="/header/anonymous.html" />
</esi:otherwise>
</esi:choose>
When the processor encounters an <esi:choose>
block, it runs through the <esi:when>
blocks, checking the expressions set in their test attributes. The processor executes the first <esi:when>
block where the expression evaluates to true. If none of the expressions evaluates to true, then it will optionally execute the <esi:otherwise>
block if it’s provided. The entire <esi:choose>
block is replaced by the entirety of whichever <esi:when>
or <esi:otherwise>
block that executes.
The processor makes available a limited set of variables, which are based primarily on request cookies. In the above example, an HTTP cookie by the name “group” is checked for its value. Our implementation is based on the ESI language specification; refer to it for more details.
List of supported tags and features
This implementation supports the following tags of the ESI language specification.
esi:include
esi:comment
esi:remove
esi:try / esi:attempt / esi:except
esi:choose / esi:when / esi:otherwise
esi:vars
The <esi:inline>
tag is defined in the spec to be optional, and is not included in this implementation.
ESI Variables are supported in the attributes of ESI tags, and ESI Expressions are supported in the test attribute of <esi:when>
. Additionally, the <!--esi ...-->
comment is supported.
Custom behavior means endless possibilities
While the feature set is enough to get thrilled about, the truly exciting part of being programmable is that even more things are possible. Bringing in templates is the main use of ESI, but that’s by no means all that it can do. Here’s an example:
Consider you have a timestamp marked up in your document that you want to be represented as a relative date when it’s displayed, such as “2 days ago”. There are many ways of doing this, but to have the best performance and memory implications, it would be great to perform a find/replace in streams. Programming this ESI library can actually be used as a good option for doing this.
We can define timestamps to be encoded in your backend document using a specially-crafted ESI tag in a format such as the following:
<esi:include src="/__since/<unix-timestamp>" />
For example, this snippet can represent midnight on January 1, 2024, Pacific time:
<h1>This document was written <esi:include src="/__since/1704034800" />.</h1>
Now, set up the EsiTransformStream
to serve a synthetic replacement document whenever it sees that URL pattern:
const esiTransformStream = new EsiTransformStream(req.url, req.headers, {
fetch(input, init) {
const url = new URL(input instanceof Request ? input.url : String(input));
// If custom URL pattern, return synthetic Response
const sinceMatch = /^\/__since\/(\d+)$/.exec(url.pathname);
if (sinceMatch != null) {
const timestampString = sinceMatch[1];
const timestamp = parseInt(timestampString, 10);
return new Response(
toRelativeTimeSince(timestamp), // This function builds a relative date
{
headers: { 'Content-Type': 'text/plain', },
status: 200,
}
);
}
// For all other esi:include tags, add the backend and call the global fetch
return fetch(input, { ...init, backend: 'origin_0' });
}
});
Now, when the processor encounters the example snippet above, it will emit a result similar to the following (depending on how many days into the future you run it):
<h1>This document was written 22d ago.</h1>
Because the backend document is cacheable by Fastly, future requests can benefit from a cache HIT, while the processing will continue to display the updated relative time.
For a live example of this, view the following fiddle:
Take it for a spin!
@fastly/esi is now available on npm, ready to be added to any JavaScript program. Use it at the edge in your Fastly Compute programs, but it can even work outside of Compute, as long as your environment supports the fetch API. The full source code is available on GitHub.
Get started by cloning either of the Fiddles above and testing them out with your own origins, even before you have created a Fastly account. When you’re ready to publish your service on our global network, you can sign up for a free trial of Compute and then get started right away with the ESI library on npm.
With Compute, the edge is programmable and modular – choose and combine the solutions that work best for you, or even build your own. We aren’t the only ones who can provide modules like this for Compute. Anyone can contribute to the ecosystem and take from it. And, as always, meet us at the Fastly community forum and let us know what you’ve been building!