Remix is a popular, modern JavaScript-based full stack web framework that allows the developer to focus on the user interface and work with web standards, in order to deliver user experiences that are responsive and robust. With our new remix-compute-js libraries, you can now host your Remix application on our Compute platform, allowing you to serve at our world-wide edge network — you don't even need an origin server.
As our support for JavaScript on Compute grows, we are increasingly able to offer more integrations to work with existing JavaScript libraries and frameworks. We talked about serving static websites entirely at the edge earlier this year, and more recently, running your Next.js site at the edge. And today, we’d like to share with you some work we’ve done to support running Remix.
Remix is a popular JavaScript-based full stack web framework with an emphasis on web standards and progressive enhancement. It’s a modern framework that fully embraces the Fetch API interfaces, making it a great fit for Compute, which does the same. And best of all, Remix has a bring-your-own-runtime design, written from the ground up to work with various server runtimes and server adapters, so that it can run on a multitude of platforms.
As a result we’ve been able to build a Remix runtime, an entry point adapter, and a project template to give you full support to target Compute as an environment to run Remix.
You now have the full power of Remix available to your Compute application, with its rich support of Web Standards and progressive enhancement. You’re free to build your Remix project as you wish, using its handy features: defining convention-based routing, harnessing the power of React Router, rendering MDX, and building API Routes.
And of course, you can take advantage of Compute features too, such as making fetch calls to backends, or using polyfills to integrate with compatible modules available from NPM.
Check out the Forrester New Wave™ for Edge Development.
Create your Remix project
A template for Fastly Compute exists on GitHub at https://github.com/fastly/remix-compute-js/tree/main/packages/remix-template. This means you just need Node.js installed locally, and then you can create a Remix project by using the official create-remix
command-line tool:
npx create-remix@latest ./my-app --template https://github.com/fastly/remix-compute-js/tree/main/packages/remix-template
You’ll be asked if you want to create your project using JavaScript or TypeScript (my personal preference is TypeScript!). The rest of the process will happen automatically.
If you switch to the ./my-app
directory, you’ll see that you’ll have a project structure built out. In addition to being a Remix project, it will also be a Compute JavaScript application complete with a fastly.toml
file that describes your application to Fastly, and an entry point at src/index.js
.
Your application is preconfigured to work on Fastly’s Development Server, which will be available just by having the Fastly CLI installed. Simply run the following command:
npm run dev
Open up http://127.0.0.1:7676 in your web browser, and you will see the Remix template application showing up.
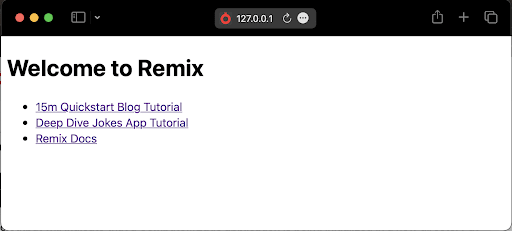
This file is built from the app/routes/index.jsx
file (.tsx
if you’re using TypeScript!). You can go ahead and make changes to this file, and you’ll see that your application will be rebuilt, and your browser window will refresh automatically.
There are actually two processes running here: Remix’s compiler and Fastly’s development server, both running in watch mode. Whenever you make changes to files in the app/
directory, the Remix compiler will trigger a rebuild, causing output files to update in the build/
and public/build/
directories. Whenever this happens, or whenever you update files in the public/
directory, Fastly’s development server will rebuild. In effect, you now have a self-refreshing development environment for your Remix application running on Compute.
The server adapter
Remix’s powerful architecture keeps two concerns separate: the Remix application itself, which is your code written within the Remix framework; and the interface between Remix and the server platform. This provides for flexibility in how the server platform interfaces with Remix - great news for platform providers like us (thanks Remix team!).
The simplest usage, which is also the one implemented in the project template, is to use the createEventHandler
function Fastly exports from the server adapter package. It expects staticAssets
, imported from the ./statics
file that is generated by the Static Publisher (also installed automatically when using the template):
import { createEventHandler } from "@fastly/remix-server-adapter";
import { staticAssets } from "./statics";
addEventListener("fetch", createEventHandler({ staticAssets }));
But if you have a case where you want more granular control over the ServerBuild
module to use with Remix, or how to handle requests for static assets, we also provide lower-level functions to help you do that:
import { createRequestHandler, handleAsset } from "@fastly/remix-server-adapter";
import { staticAssets } from "./statics";
const build = staticAssets.getAsset("/build/index.js").module;
const requestHandler = createRequestHandler({build});
addEventListener("fetch", (event) => event.respondWith(handleRequest(event)));
async function handleRequest(event) {
let response = await handleAsset(event, staticAssets);
if (!response) {
response = requestHandler(event);
}
return response;
}
Going Live
When you're ready to ship, create a production build and view your site to make sure that it runs well.
Stop the development server, then run the following commands:
npm run build
npm start
This generates a production build of your application, generating optimized JavaScript files. Then refresh your browser to see the production-build version of your app running in the development server (live reloading is not available in production builds).
Once you’ve seen that the production build of your site looks good, you're ready to deploy to Fastly. If you don't already have an account, start by creating one and then configure Fastly CLI with your new account credentials. Once that's done, you can deploy your app to your Fastly account:
npm run build
npm run deploy
You'll be prompted to create a new Fastly service. If you want to use an existing service, modify fastly.toml
and set the service_id
value to your service ID before running the commands above.
And that’s really all there is to using Remix on Compute: Develop as normal using npm run dev
. Test with npm run build
and npm start
, and when you’re ready to go to production, deploy to the edge with npm run build
and npm run deploy
.
Already have a Remix application? Let’s bring it to Compute
Do you already have a Remix application that you’ve started? Perhaps one already hosted on a Node.js backend? So long as your project doesn’t attempt to do something that isn’t conceptually impossible on Fastly, such as trying to interact with the filesystem, it’s usually not too hard to adapt the application to work on Compute.
The easiest way to do this is to create an empty Remix project using the template above. That way, all of the dependencies will be installed for you, and the server adapter will be created as part of the process as well. Install into your new project any dependency packages referenced by your original project. Then, move your existing project’s files into your new project. In Remix, your application’s files are found in app/ and public/. For most of the files in these directories, you can usually simply copy them into your new project, keeping the following points in mind.
1. Some of the source files will reference Remix runtime packages such as @remix-run/node
, @remix-run/cloudflare
, or @remix-run/deno
. Modify these references to @fastly/remix-server-runtime
.
For example, you may have the following:
import { json } from "@remix-run/node";
You’ll want to change this to:
import { json } from "@fastly/remix-server-runtime";
2. For entry.client.tsx
, entry.server.tsx
, and root.tsx
in the app/
directory, you’ll generally want to start with the ones found in the new project. Examine these files in your source project. Look for any changes that you had made to them in your source project, and then make the equivalent changes in the new project as necessary.
3. Skip /public/build/
. This is a directory that is generated during the build step.
4. Depending on the dependencies used by your project, you may need to add polyfills to run in Compute.
And that’s all there is to it! Build and deploy your application to the edge as we did in the previous section.
Your Remix application, now on Compute
The integration between Remix and Compute is here – you can now host your Remix application entirely at the edge, without even needing an origin server. Benefit from the robust architecture of Remix, and then go to Compute to run your app on thousands of servers across Fastly's network.
At Fastly, we strive to provide the tools that give you the power to run more code at the Edge and develop for it with the tools you know and love. We love to hear it when our users get the most out of these tools. Reach out to us on Twitter and let us know what you’ve been building!